Disk management in vCenter is a straightforward process. However, what if we want to create a new disk using a code? What if we would like to specify a storage controller to which we aim to attach the disk? What if we intend to ensure that the storage controller has a free slot to attach a new disk? Today, we will attempt to accomplish these tasks. While the relatively simple task may seem straightforward, it will turn out to be slightly more challenging than it initially appears.
General goals
- Show the user all necessary details.
- Support for all available storage controllers.
- Validate that there are available slots in the storage controller to attach a new disk and warn the user.
- Limit the maximum disk size.
- Automatically find the next available slot in a selected storage controller.
vRBT goals:
- Explore how to create multiple scriptable tasks with vRBT
To achieve our objectives, we’ll need to gather some specific details based on the provided user information and collect data directly from the virtual machine.
A use case:
We aim to create and attach a new disk with a custom size to one of the available storage controllers within the virtual machine:
- Ask the user to select a VM.
- Ask the user to select one of the available storage controllers.
- Ask the user to provide a disk size.
- Run the workflow.
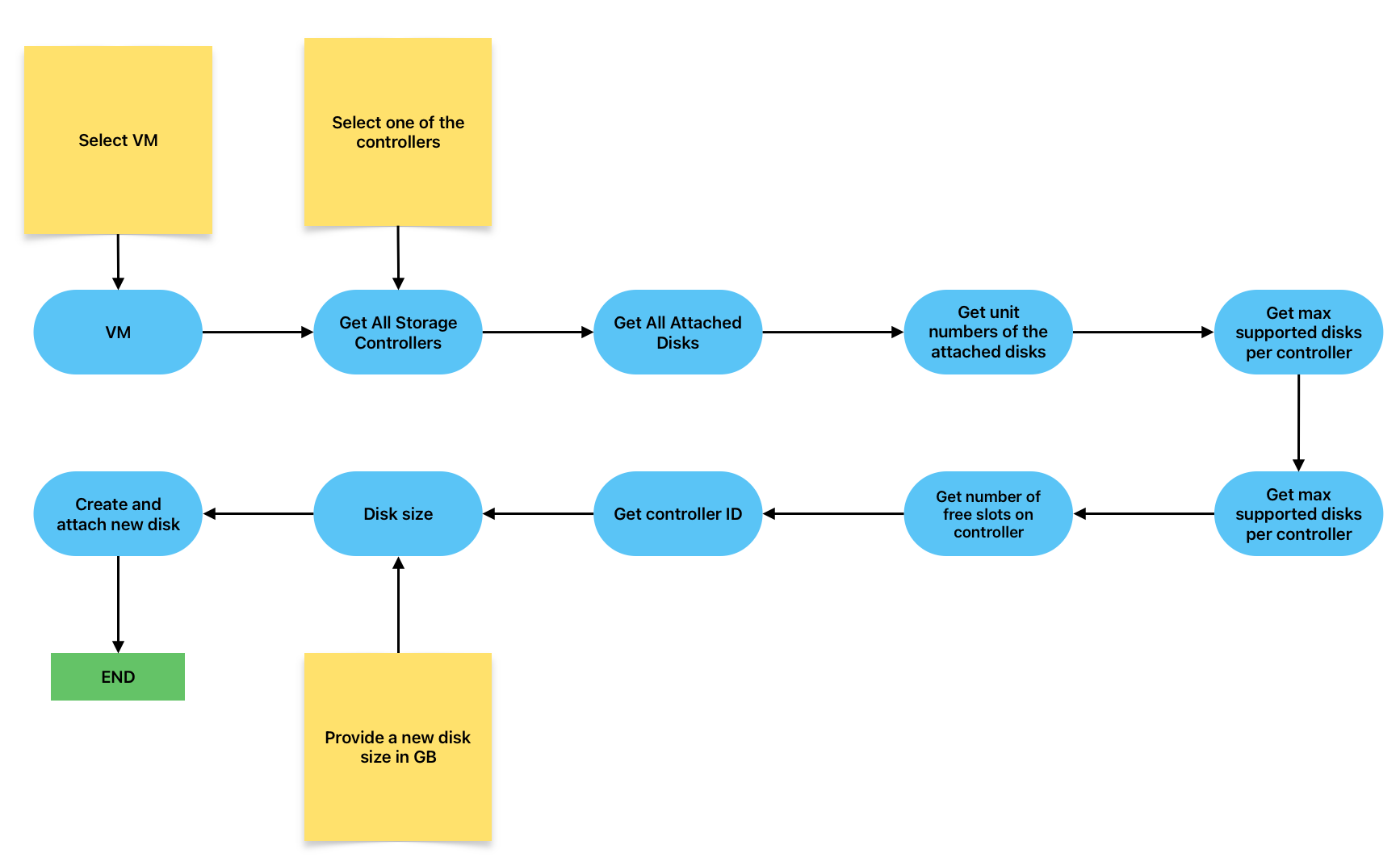
The solution
Get all available storage controller names
To display the list of all available storage controllers in the selected virtual machine on a custom form, we need to create a function called getDeviceControllerNames
. This function is straightforward and retrieves an array of storage controller names by invoking the getDeviceControllers
function.
/**
* Get the device controllers names
*
* @param {VC:VirtualMachine} vm - The name of the virtual machine to check.
* @returns {Array/string} - Array of used device controllers names
*/
(function (vm) {
if (!vm) return [];
var deviceControllers = System.getModule(
"com.clouddepth.disk_management.actions"
).getDeviceControllers(vm);
return deviceControllers.map(function (device) {
return device.deviceInfo.label;
});
});
The getDeviceControllers
function queries all available types of controllers in the virtual machine and returns only those that are instances of a specific type.
/**
* Get the device controllers associated
*
* @param {VC:VirtualMachine} vm - The name of the virtual machine to check.
* @returns {Array/object} - Array of used device controllers associated
*/
(function (vm) {
if (!vm) return [];
var devices = vm.config.hardware.device;
return devices.filter(function (device) {
return (
device instanceof VcParaVirtualSCSIController ||
device instanceof VcVirtualIDEController ||
device instanceof VcVirtualAHCIController ||
device instanceof VcVirtualLsiLogicSASController ||
device instanceof VcVirtualNVMEController
);
});
});
As a result, we have this list below.
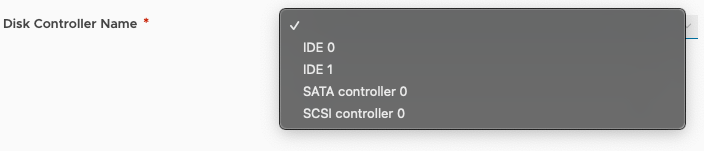